
在 Vue 3 中,自定义 Hook 通常用于封装可复用的逻辑,并且通常不直接访问组件实例的上下文(如 props
、emit
、slots
等)。然而,如果你需要在自定义 Hook 中访问组件实例的上下文,可以通过将上下文作为参数传递给自定义 Hook 来实现。
1. 传递 props
和 emit
你可以将 props
和 emit
作为参数传递给自定义 Hook,以便在 Hook 内部访问它们。
// useCustomHook.js
import { ref, watch } from 'vue';
export function useCustomHook(props, emit) {
const count = ref(0);
watch(() => props.initialCount, (newValue) => {
count.value = newValue;
});
function increment() {
count.value++;
emit('count-updated', count.value);
}
return {
count,
increment
};
}
2. 在组件中使用自定义 Hook
在组件中,你可以将 props
和 emit
传递给自定义 Hook。
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script>
import { useCustomHook } from './useCustomHook';
export default {
props: {
initialCount: {
type: Number,
default: 0
}
},
emits: ['count-updated'],
setup(props, { emit }) {
const { count, increment } = useCustomHook(props, emit);
return {
count,
increment
};
}
};
</script>
3. 在 <script setup>
语法中使用自定义 Hook
在 <script setup>
语法中,你可以使用 defineProps
和 defineEmits
来定义 props
和 emit
,并将它们传递给自定义 Hook。
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script setup>
import { useCustomHook } from './useCustomHook';
const props = defineProps({
initialCount: {
type: Number,
default: 0
}
});
const emit = defineEmits(['count-updated']);
const { count, increment } = useCustomHook(props, emit);
</script>
4. 访问 slots
和 attrs
如果你需要访问 slots
或 attrs
,可以将它们作为参数传递给自定义 Hook。
// useCustomHook.js
import { ref, watch } from 'vue';
export function useCustomHook(props, emit, slots, attrs) {
const count = ref(0);
watch(() => props.initialCount, (newValue) => {
count.value = newValue;
});
function increment() {
count.value++;
emit('count-updated', count.value);
}
return {
count,
increment,
slots,
attrs
};
}
5. 在组件中使用自定义 Hook 并访问 slots
和 attrs
在组件中,你可以将 slots
和 attrs
传递给自定义 Hook。
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="increment">Increment</button>
<div v-if="slots.default">
<slot></slot>
</div>
<div v-if="attrs.customAttr">
Custom Attribute: {{ attrs.customAttr }}
</div>
</div>
</template>
<script>
import { useCustomHook } from './useCustomHook';
export default {
props: {
initialCount: {
type: Number,
default: 0
}
},
emits: ['count-updated'],
setup(props, { emit, slots, attrs }) {
const { count, increment, slots: hookSlots, attrs: hookAttrs } = useCustomHook(props, emit, slots, attrs);
return {
count,
increment,
slots: hookSlots,
attrs: hookAttrs
};
}
};
</script>
6. 在 <script setup>
语法中使用自定义 Hook 并访问 slots
和 attrs
在 <script setup>
语法中,你可以使用 useSlots
和 useAttrs
来访问 slots
和 attrs
,并将它们传递给自定义 Hook。
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="increment">Increment</button>
<div v-if="slots.default">
<slot></slot>
</div>
<div v-if="attrs.customAttr">
Custom Attribute: {{ attrs.customAttr }}
</div>
</div>
</template>
<script setup>
import { useCustomHook } from './useCustomHook';
import { useSlots, useAttrs } from 'vue';
const props = defineProps({
initialCount: {
type: Number,
default: 0
}
});
const emit = defineEmits(['count-updated']);
const slots = useSlots();
const attrs = useAttrs();
const { count, increment } = useCustomHook(props, emit, slots, attrs);
</script>
总结
- 在自定义 Hook 中,可以通过将
props
、emit
、slots
和attrs
作为参数传递来访问组件实例的上下文。 - 在组件中,你可以将这些上下文传递给自定义 Hook,并在 Hook 内部使用它们。
- 在
<script setup>
语法中,可以使用defineProps
、defineEmits
、useSlots
和useAttrs
来访问组件实例的上下文,并将它们传递给自定义 Hook。 - 通过这种方式,你可以在自定义 Hook 中访问组件实例的上下文,同时保持逻辑的可复用性和封装性。
学在每日,进无止境!更多精彩内容请关注微信公众号。
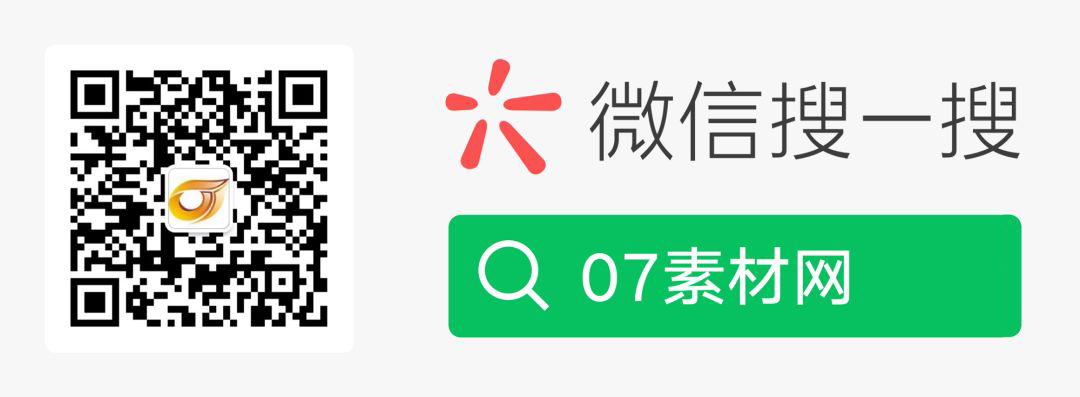
原文出处:
内容由AI生成仅供参考,请勿使用于商业用途。如若转载请注明原文及出处。
出处地址:http://www.07sucai.com/tech/873.html
版权声明:本文来源地址若非本站均为转载,若侵害到您的权利,请及时联系我们,我们会在第一时间进行处理。