
测试自定义 Hook 是确保其逻辑正确性和稳定性的重要步骤。由于自定义 Hook 通常是基于 Vue 的组合式 API 构建的,因此可以使用 Vue Test Utils 和 Jest 等工具来测试它们。以下是如何测试自定义 Hook 的详细步骤和示例:
1. 设置测试环境
首先,确保你已经安装了必要的测试工具:
- Vue Test Utils: 用于测试 Vue 组件的工具。
- Jest: 用于编写和运行测试的 JavaScript 测试框架。
你可以通过以下命令安装这些工具:
npm install --save-dev @vue/test-utils jest
2. 创建自定义 Hook
假设你有一个自定义 Hook useCounter
,用于管理计数器的状态和逻辑。
// useCounter.js
import { ref } from 'vue';
export function useCounter(initialValue = 0) {
const count = ref(initialValue);
function increment() {
count.value++;
}
function decrement() {
count.value--;
}
return {
count,
increment,
decrement
};
}
3. 编写测试用例
创建一个测试文件 useCounter.spec.js
,并编写测试用例来验证 useCounter
的行为。
// useCounter.spec.js
import { useCounter } from './useCounter';
import { ref } from 'vue';
describe('useCounter', () => {
it('should initialize with the correct initial value', () => {
const { count } = useCounter(10);
expect(count.value).toBe(10);
});
it('should increment the count', () => {
const { count, increment } = useCounter(0);
increment();
expect(count.value).toBe(1);
});
it('should decrement the count', () => {
const { count, decrement } = useCounter(1);
decrement();
expect(count.value).toBe(0);
});
});
4. 运行测试
使用 Jest 运行测试:
npx jest
如果一切正常,你应该会看到测试通过的输出。
5. 测试带有副作用的 Hook
如果自定义 Hook 包含副作用(如定时器、异步请求等),可以使用 Jest 的 jest.useFakeTimers
和 jest.runAllTimers
来模拟和控制时间。
// useInterval.js
import { ref, onUnmounted } from 'vue';
export function useInterval(callback, delay) {
const intervalId = ref(null);
intervalId.value = setInterval(callback, delay);
onUnmounted(() => {
clearInterval(intervalId.value);
});
return intervalId;
}
编写测试用例:
// useInterval.spec.js
import { useInterval } from './useInterval';
import { onUnmounted } from 'vue';
describe('useInterval', () => {
beforeEach(() => {
jest.useFakeTimers();
});
afterEach(() => {
jest.useRealTimers();
});
it('should call the callback at the specified interval', () => {
const callback = jest.fn();
useInterval(callback, 1000);
jest.advanceTimersByTime(1000);
expect(callback).toHaveBeenCalledTimes(1);
jest.advanceTimersByTime(1000);
expect(callback).toHaveBeenCalledTimes(2);
});
it('should clear the interval when the component is unmounted', () => {
const callback = jest.fn();
const { intervalId } = useInterval(callback, 1000);
onUnmounted(() => {
clearInterval(intervalId.value);
});
jest.advanceTimersByTime(1000);
expect(callback).toHaveBeenCalledTimes(1);
onUnmounted();
jest.advanceTimersByTime(1000);
expect(callback).toHaveBeenCalledTimes(1); // Callback should not be called again
});
});
6. 测试异步逻辑
如果自定义 Hook 包含异步逻辑(如 API 请求),可以使用 Jest 的 jest.mock
来模拟异步请求。
// useFetch.js
import { ref } from 'vue';
export function useFetch(url) {
const data = ref(null);
const error = ref(null);
const loading = ref(false);
async function fetchData() {
loading.value = true;
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error('Network response was not ok');
}
data.value = await response.json();
} catch (err) {
error.value = err;
} finally {
loading.value = false;
}
}
fetchData();
return { data, error, loading };
}
编写测试用例:
// useFetch.spec.js
import { useFetch } from './useFetch';
import { ref } from 'vue';
global.fetch = jest.fn(() =>
Promise.resolve({
ok: true,
json: () => Promise.resolve({ data: 'mock data' }),
})
);
describe('useFetch', () => {
it('should fetch data successfully', async () => {
const { data, error, loading } = useFetch('https://api.example.com/data');
await Promise.resolve(); // Wait for the next tick
expect(loading.value).toBe(false);
expect(data.value).toEqual({ data: 'mock data' });
expect(error.value).toBeNull();
});
it('should handle fetch error', async () => {
global.fetch.mockImplementationOnce(() =>
Promise.reject(new Error('Network error'))
);
const { data, error, loading } = useFetch('https://api.example.com/data');
await Promise.resolve(); // Wait for the next tick
expect(loading.value).toBe(false);
expect(data.value).toBeNull();
expect(error.value).toEqual(new Error('Network error'));
});
});
7. 测试组件中使用自定义 Hook
如果你在组件中使用了自定义 Hook,可以使用 Vue Test Utils 来测试组件的行为。
// CounterComponent.vue
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script>
import { useCounter } from './useCounter';
export default {
setup() {
const { count, increment } = useCounter(0);
return {
count,
increment
};
}
};
</script>
编写测试用例:
// CounterComponent.spec.js
import { mount } from '@vue/test-utils';
import CounterComponent from './CounterComponent.vue';
describe('CounterComponent', () => {
it('should increment the count when the button is clicked', async () => {
const wrapper = mount(CounterComponent);
expect(wrapper.text()).toContain('Count: 0');
await wrapper.find('button').trigger('click');
expect(wrapper.text()).toContain('Count: 1');
});
});
总结
- 使用 Jest 和 Vue Test Utils 来测试自定义 Hook。
- 对于简单的逻辑,可以直接测试 Hook 的返回值。
- 对于包含副作用的 Hook,可以使用 Jest 的
jest.useFakeTimers
和jest.runAllTimers
来模拟和控制时间。 - 对于异步逻辑,可以使用
jest.mock
来模拟异步请求。 - 对于组件中使用自定义 Hook 的情况,可以使用 Vue Test Utils 来测试组件的行为。
- 通过编写全面的测试用例,可以确保自定义 Hook 的逻辑正确性和稳定性。
学在每日,进无止境!更多精彩内容请关注微信公众号。
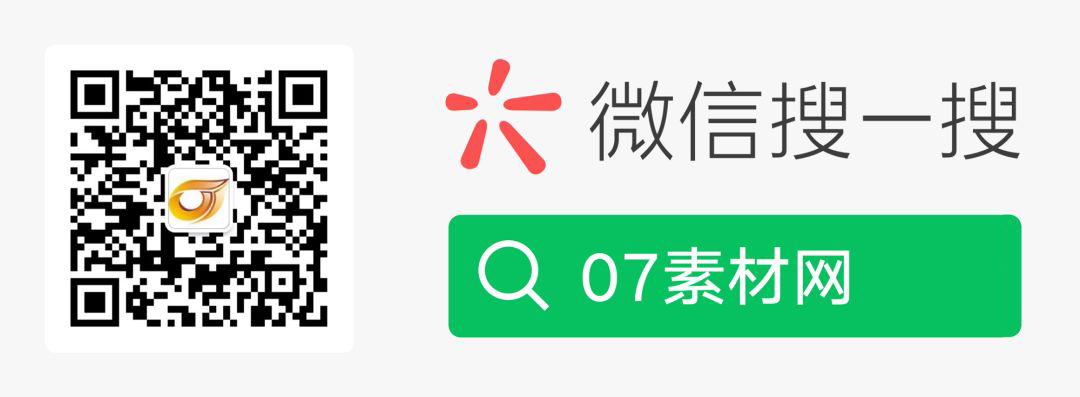
原文出处:
内容由AI生成仅供参考,请勿使用于商业用途。如若转载请注明原文及出处。
出处地址:http://www.07sucai.com/tech/874.html
版权声明:本文来源地址若非本站均为转载,若侵害到您的权利,请及时联系我们,我们会在第一时间进行处理。