
在大型应用中,状态管理可能会变得复杂,Pinia 提供了多种机制来有效地处理这种复杂性。以下是如何在大型应用中使用 Pinia 进行状态管理的最佳实践:
1. 模块化 Store
将应用的状态拆分为多个独立的 Store,每个 Store 负责管理特定的功能模块。这种模块化的设计使得状态管理更加清晰和可维护。
示例:模块化 Store
// stores/user.ts
import { defineStore } from 'pinia';
export const useUserStore = defineStore('user', {
state: () => ({
users: [] as any[],
currentUser: null as any,
}),
actions: {
async fetchUsers() {
const response = await fetch('/api/users');
this.users = await response.json();
},
async fetchUser(userId: number) {
const response = await fetch(`/api/users/${userId}`);
this.currentUser = await response.json();
},
},
});
// stores/product.ts
import { defineStore } from 'pinia';
export const useProductStore = defineStore('product', {
state: () => ({
products: [] as any[],
currentProduct: null as any,
}),
actions: {
async fetchProducts() {
const response = await fetch('/api/products');
this.products = await response.json();
},
async fetchProduct(productId: number) {
const response = await fetch(`/api/products/${productId}`);
this.currentProduct = await response.json();
},
},
});
2. 按需加载 Store
在大型应用中,不是所有的 Store 都需要在应用启动时加载。可以使用动态导入(dynamic import)按需加载 Store,减少初始加载时的资源消耗。
示例:按需加载 Store
// 在组件中动态加载 Store
import { defineAsyncComponent } from 'vue';
const UserProfile = defineAsyncComponent(() =>
import('@/stores/userProfile').then((module) => module.useUserProfileStore())
);
3. 共享状态和逻辑
如果多个 Store 需要共享状态或逻辑,可以创建一个共享的 Store 或使用插件机制。
示例:共享状态
// stores/shared.ts
import { defineStore } from 'pinia';
export const useSharedStore = defineStore('shared', {
state: () => ({
loading: false,
error: null as string | null,
}),
actions: {
setLoading(loading: boolean) {
this.loading = loading;
},
setError(error: string | null) {
this.error = error;
},
},
});
在其他 Store 中使用共享状态
// stores/user.ts
import { defineStore } from 'pinia';
import { useSharedStore } from './shared';
export const useUserStore = defineStore('user', {
actions: {
async fetchUsers() {
const sharedStore = useSharedStore();
sharedStore.setLoading(true);
try {
const response = await fetch('/api/users');
this.users = await response.json();
} catch (error) {
sharedStore.setError(error instanceof Error ? error.message : 'Unknown error');
} finally {
sharedStore.setLoading(false);
}
},
},
});
4. 使用插件扩展功能
Pinia 支持插件机制,可以通过插件为 Store 添加全局功能或共享逻辑。
示例:日志插件
// plugins/logger.ts
import { PiniaPluginContext } from 'pinia';
export function loggerPlugin(context: PiniaPluginContext) {
context.store.$subscribe((mutation, state) => {
console.log(`Store ${context.store.$id} changed:`, state);
});
}
// 使用插件
import { createPinia } from 'pinia';
import { loggerPlugin } from './plugins/logger';
const pinia = createPinia();
pinia.use(loggerPlugin);
5. 优化性能
在大型应用中,性能优化非常重要。可以通过以下方式优化 Pinia 的性能:
- 按需加载 Store:减少初始加载时的资源消耗。
- 减少不必要的状态更新:使用
storeToRefs
和computed
。 - 使用缓存:减少重复请求和数据计算。
- 避免频繁的状态更新:使用防抖(debounce)或节流(throttle)技术。
示例:使用防抖
import { defineStore } from 'pinia';
import { debounce } from 'lodash-es';
export const useScrollStore = defineStore('scroll', {
state: () => ({
scrollPosition: 0,
}),
actions: {
updateScrollPosition: debounce(function (this: any, position: number) {
this.scrollPosition = position;
}, 100), // 100ms 防抖
},
});
6. 使用 TypeScript 增强类型安全
在大型应用中,类型安全非常重要。Pinia 原生支持 TypeScript,可以为 Store 的状态、getters 和 actions 添加类型注解,确保类型安全。
示例:使用 TypeScript
// stores/user.ts
import { defineStore } from 'pinia';
interface User {
id: number;
name: string;
email: string;
}
export const useUserStore = defineStore('user', {
state: () => ({
users: [] as User[],
currentUser: null as User | null,
}),
actions: {
async fetchUsers() {
const response = await fetch('/api/users');
this.users = await response.json();
},
async fetchUser(userId: number) {
const response = await fetch(`/api/users/${userId}`);
this.currentUser = await response.json();
},
},
});
7. 处理跨 Store 的依赖
如果多个 Store 之间存在依赖关系,可以在一个 Store 中导入并使用另一个 Store。
示例:跨 Store 依赖
// stores/user.ts
import { defineStore } from 'pinia';
import { useProductStore } from './product';
export const useUserStore = defineStore('user', {
actions: {
async fetchUserWithProducts(userId: number) {
const productStore = useProductStore();
await this.fetchUser(userId);
await productStore.fetchProducts();
},
},
});
总结
在大型应用中使用 Pinia 进行状态管理的最佳实践包括:
- 模块化 Store:将状态拆分为多个独立的 Store。
- 按需加载 Store:减少初始加载时的资源消耗。
- 共享状态和逻辑:通过共享 Store 或插件机制实现。
- 使用插件扩展功能:为 Store 添加全局功能或共享逻辑。
- 优化性能:按需加载、减少不必要的更新、使用缓存和防抖技术。
- 使用 TypeScript 增强类型安全:确保状态管理的类型安全。
- 处理跨 Store 的依赖:在一个 Store 中导入并使用另一个 Store。
通过这些方法,Pinia 能够有效地处理大型应用中的状态管理,确保代码的可维护性和性能。
学在每日,进无止境!更多精彩内容请关注微信公众号。
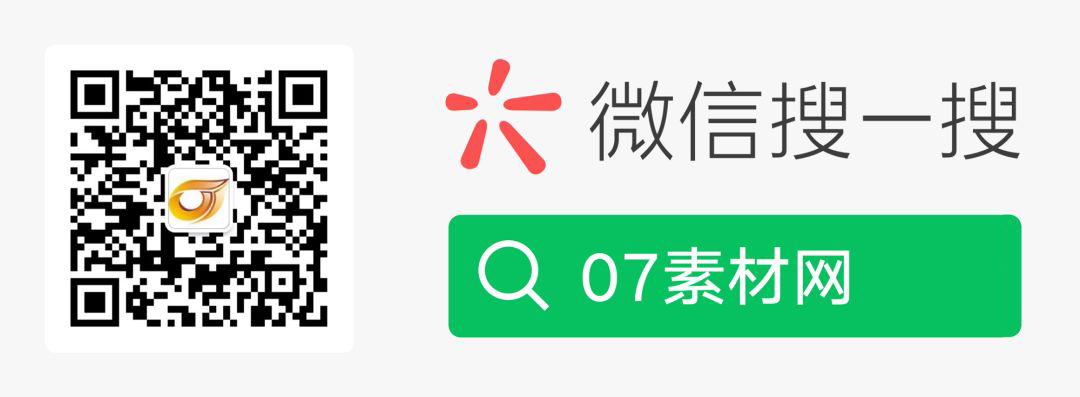
原文出处:
内容由AI生成仅供参考,请勿使用于商业用途。如若转载请注明原文及出处。
出处地址:http://www.07sucai.com/tech/839.html
版权声明:本文来源地址若非本站均为转载,若侵害到您的权利,请及时联系我们,我们会在第一时间进行处理。