
在 Vue 3 中,watchEffect
是用于监听响应式数据变化的工具。与 watch
不同,watchEffect
不需要显式指定要监听的数据源,它会自动追踪回调函数中使用的所有响应式数据,并在这些数据变化时重新执行回调函数。以下是使用 watchEffect
监听数据变化的详细步骤和示例:
1. 引入 watchEffect
首先,你需要在 Vue 组件中引入 watchEffect
函数。
import { watchEffect } from 'vue';
2. 创建 watchEffect
使用 watchEffect
创建一个监听器,传入一个回调函数。watchEffect
会立即执行该回调函数,并自动追踪回调函数中使用的所有响应式数据。
const count = ref(0);
watchEffect(() => {
console.log(`count is: ${count.value}`);
});
3. 在模板中使用
在模板中,你可以直接使用 watchEffect
监听的数据。
<template>
<div>
<p>{{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script>
import { ref, watchEffect } from 'vue';
export default {
setup() {
const count = ref(0);
watchEffect(() => {
console.log(`count is: ${count.value}`);
});
function increment() {
count.value++;
}
return {
count,
increment
};
}
};
</script>
4. 在组合式 API 中使用
在组合式 API 中,watchEffect
通常用于在 setup
函数中监听响应式数据的变化。
import { ref, watchEffect } from 'vue';
export default {
setup() {
const count = ref(0);
watchEffect(() => {
console.log(`count is: ${count.value}`);
});
function increment() {
count.value++;
}
return {
count,
increment
};
}
};
5. 在 <script setup>
语法中使用
如果你使用 <script setup>
语法,可以更简洁地使用 watchEffect
。
<template>
<div>
<p>{{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script setup>
import { ref, watchEffect } from 'vue';
const count = ref(0);
watchEffect(() => {
console.log(`count is: ${count.value}`);
});
function increment() {
count.value++;
}
</script>
6. 停止监听
watchEffect
返回一个停止监听的函数,调用该函数可以停止监听。
const stopWatchEffect = watchEffect(() => {
console.log(`count is: ${count.value}`);
});
// 停止监听
stopWatchEffect();
7. 清理副作用
watchEffect
的回调函数可以接收一个 onInvalidate
函数,用于清理副作用(如取消请求、清除定时器等)。
watchEffect((onInvalidate) => {
const timer = setTimeout(() => {
console.log(`count is: ${count.value}`);
}, 1000);
onInvalidate(() => {
clearTimeout(timer);
});
});
8. 监听多个响应式数据
watchEffect
会自动追踪回调函数中使用的所有响应式数据,并在这些数据变化时重新执行回调函数。
const count = ref(0);
const name = ref('Vue 3');
watchEffect(() => {
console.log(`count is: ${count.value}, name is: ${name.value}`);
});
9. 在模板中使用多个响应式数据
在模板中,你可以直接使用 watchEffect
监听的多个响应式数据。
<template>
<div>
<p>{{ count }}</p>
<p>{{ name }}</p>
<button @click="increment">Increment</button>
<button @click="changeName">Change Name</button>
</div>
</template>
<script setup>
import { ref, watchEffect } from 'vue';
const count = ref(0);
const name = ref('Vue 3');
watchEffect(() => {
console.log(`count is: ${count.value}, name is: ${name.value}`);
});
function increment() {
count.value++;
}
function changeName() {
name.value = 'Vue 3 is awesome!';
}
</script>
10. 处理异步操作
watchEffect
可以处理异步操作,并在依赖的响应式数据变化时重新执行。
const count = ref(0);
watchEffect(async (onInvalidate) => {
const data = await fetchData(count.value);
onInvalidate(() => {
// 清理操作
});
console.log('Fetched data:', data);
});
总结
- 使用
watchEffect
监听响应式数据变化时,传入一个回调函数,watchEffect
会自动追踪回调函数中使用的所有响应式数据。 watchEffect
会立即执行回调函数,并在依赖的响应式数据变化时重新执行。watchEffect
返回一个停止监听的函数,调用该函数可以停止监听。- 可以使用
onInvalidate
函数清理副作用。 - 在组合式 API 和
<script setup>
语法中,watchEffect
是监听响应式数据变化的常用工具。
学在每日,进无止境!更多精彩内容请关注微信公众号。
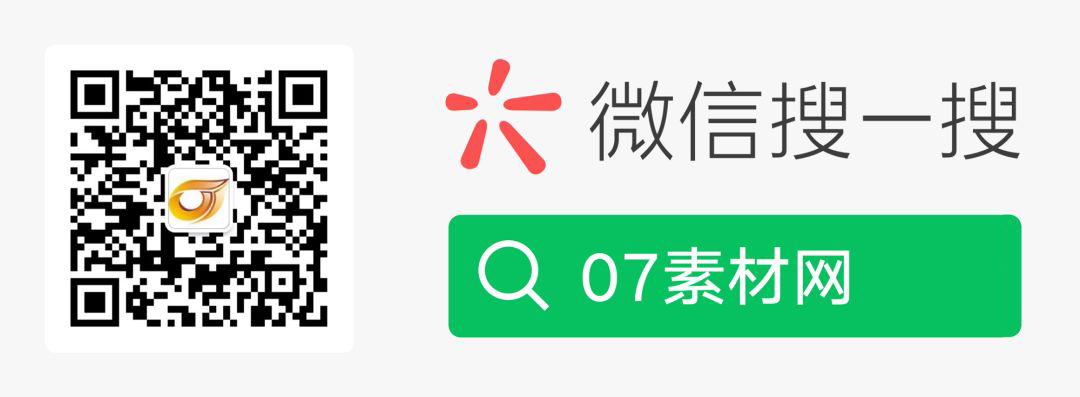
原文出处:
内容由AI生成仅供参考,请勿使用于商业用途。如若转载请注明原文及出处。
出处地址:http://www.07sucai.com/tech/867.html
版权声明:本文来源地址若非本站均为转载,若侵害到您的权利,请及时联系我们,我们会在第一时间进行处理。