
在 Vue 3 中,watch
是用于监听响应式数据变化的工具。当被监听的数据发生变化时,watch
会执行指定的回调函数。以下是使用 watch
监听数据变化的详细步骤和示例:
1. 引入 watch
首先,你需要在 Vue 组件中引入 watch
函数。
import { watch } from 'vue';
2. 监听单个数据源
使用 watch
监听一个响应式数据源(如 ref
或 reactive
对象的属性)。
const count = ref(0);
watch(count, (newValue, oldValue) => {
console.log(`count changed from ${oldValue} to ${newValue}`);
});
3. 监听多个数据源
使用 watch
监听多个响应式数据源,传入一个数组作为第一个参数。
const count = ref(0);
const name = ref('Vue 3');
watch([count, name], ([newCount, newName], [oldCount, oldName]) => {
console.log(`count changed from ${oldCount} to ${newCount}`);
console.log(`name changed from ${oldName} to ${newName}`);
});
4. 监听 reactive
对象的属性
使用 watch
监听 reactive
对象的属性时,需要传入一个返回该属性的函数。
const state = reactive({
count: 0,
name: 'Vue 3'
});
watch(() => state.count, (newValue, oldValue) => {
console.log(`count changed from ${oldValue} to ${newValue}`);
});
5. 立即执行回调
默认情况下,watch
不会立即执行回调函数。可以通过设置 immediate: true
来立即执行回调。
watch(count, (newValue, oldValue) => {
console.log(`count changed from ${oldValue} to ${newValue}`);
}, { immediate: true });
6. 深度监听
当监听一个对象或数组时,默认情况下 watch
不会深度监听嵌套属性的变化。可以通过设置 deep: true
来启用深度监听。
const state = reactive({
user: {
name: 'John',
age: 30
}
});
watch(() => state.user, (newValue, oldValue) => {
console.log('user changed', newValue);
}, { deep: true });
7. 停止监听
watch
返回一个停止监听的函数,调用该函数可以停止监听。
const stopWatch = watch(count, (newValue, oldValue) => {
console.log(`count changed from ${oldValue} to ${newValue}`);
});
// 停止监听
stopWatch();
8. 在组合式 API 中使用
在组合式 API 中,watch
通常用于在 setup
函数中监听响应式数据的变化。
import { ref, watch } from 'vue';
export default {
setup() {
const count = ref(0);
watch(count, (newValue, oldValue) => {
console.log(`count changed from ${oldValue} to ${newValue}`);
});
return {
count
};
}
};
9. 在 <script setup>
语法中使用
如果你使用 <script setup>
语法,可以更简洁地使用 watch
。
<template>
<div>
<p>{{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script setup>
import { ref, watch } from 'vue';
const count = ref(0);
watch(count, (newValue, oldValue) => {
console.log(`count changed from ${oldValue} to ${newValue}`);
});
function increment() {
count.value++;
}
</script>
10. 监听多个数据源并立即执行
你可以同时监听多个数据源,并立即执行回调函数。
const count = ref(0);
const name = ref('Vue 3');
watch([count, name], ([newCount, newName], [oldCount, oldName]) => {
console.log(`count changed from ${oldCount} to ${newCount}`);
console.log(`name changed from ${oldName} to ${newName}`);
}, { immediate: true });
11. 监听 reactive
对象的多个属性
你可以监听 reactive
对象的多个属性,并立即执行回调函数。
const state = reactive({
count: 0,
name: 'Vue 3'
});
watch(() => [state.count, state.name], ([newCount, newName], [oldCount, oldName]) => {
console.log(`count changed from ${oldCount} to ${newCount}`);
console.log(`name changed from ${oldName} to ${newName}`);
}, { immediate: true });
总结
- 使用
watch
监听响应式数据变化时,传入要监听的数据源和回调函数。 - 可以监听单个数据源、多个数据源、
reactive
对象的属性等。 - 通过设置
immediate: true
可以立即执行回调函数。 - 通过设置
deep: true
可以启用深度监听。 watch
返回一个停止监听的函数,调用该函数可以停止监听。- 在组合式 API 和
<script setup>
语法中,watch
是监听响应式数据变化的常用工具。
学在每日,进无止境!更多精彩内容请关注微信公众号。
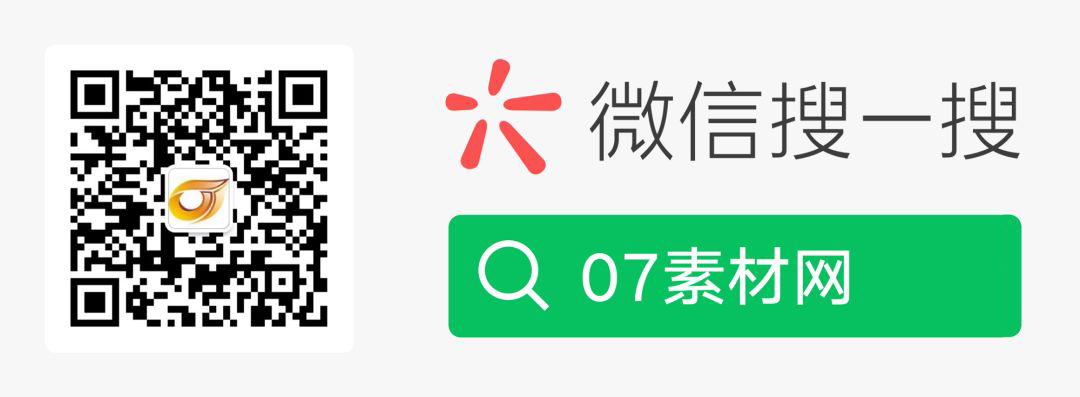
原文出处:
内容由AI生成仅供参考,请勿使用于商业用途。如若转载请注明原文及出处。
出处地址:http://www.07sucai.com/tech/866.html
版权声明:本文来源地址若非本站均为转载,若侵害到您的权利,请及时联系我们,我们会在第一时间进行处理。