Teleport
是 Vue 3 中引入的一个新特性,它允许你将组件的一部分内容“传送”到 DOM 中的其他位置。这在处理模态框、弹出框、通知等需要将内容渲染到 DOM 树中不同位置的场景时非常有用。
为什么需要 Teleport
?
在 Vue 2 中,组件的模板结构通常与 DOM 结构紧密耦合。这意味着组件的 HTML 结构必须位于其父组件的 DOM 结构中。然而,在某些情况下(如模态框、弹出框等),我们希望将组件的某些内容渲染到 DOM 树的其他位置(例如 body
元素的末尾),以避免 CSS 或 z-index 的问题。
Teleport
提供了一种简单的方式来实现这一点,使得你可以将组件的某部分内容“传送”到 DOM 中的任何位置,而不必改变组件的逻辑结构。
如何使用 Teleport
?
Teleport
的使用非常简单,你只需要在模板中使用 <teleport>
标签,并指定一个目标位置(通过 to
属性)。to
属性可以是一个 CSS 选择器字符串,也可以是一个 DOM 元素。
基本用法
<template>
<button @click="showModal = true">Show Modal</button>
<teleport to="body">
<div v-if="showModal" class="modal">
<p>This is a modal!</p>
<button @click="showModal = false">Close</button>
</div>
</teleport>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const showModal = ref(false);
return {
showModal,
};
},
};
</script>
<style>
.modal {
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background: white;
padding: 20px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
</style>
在这个例子中,当用户点击“Show Modal”按钮时,模态框会被渲染到 body
元素的末尾,而不是当前组件的 DOM 结构中。这样可以避免模态框受到父组件样式或布局的影响。
动态目标
to
属性不仅可以是一个静态的 CSS 选择器,还可以是一个动态的 DOM 元素。例如:
<template>
<button @click="showModal = true">Show Modal</button>
<teleport :to="targetElement">
<div v-if="showModal" class="modal">
<p>This is a modal!</p>
<button @click="showModal = false">Close</button>
</div>
</teleport>
</template>
<script>
import { ref, onMounted } from 'vue';
export default {
setup() {
const showModal = ref(false);
const targetElement = ref(null);
onMounted(() => {
targetElement.value = document.getElementById('modal-container');
});
return {
showModal,
targetElement,
};
},
};
</script>
<style>
.modal {
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background: white;
padding: 20px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
</style>
在这个例子中,to
属性绑定到一个动态的 DOM 元素 targetElement
,该元素在组件挂载后通过 onMounted
钩子设置为 #modal-container
。
多个 Teleport
目标
你可以在同一个组件中使用多个 <teleport>
,并将它们传送到不同的目标位置。Vue 会按照它们在模板中的顺序依次处理。
<template>
<button @click="showModal = true">Show Modal</button>
<teleport to="#modal-container-1">
<div v-if="showModal" class="modal">
<p>This is modal 1!</p>
<button @click="showModal = false">Close</button>
</div>
</teleport>
<teleport to="#modal-container-2">
<div v-if="showModal" class="modal">
<p>This is modal 2!</p>
<button @click="showModal = false">Close</button>
</div>
</teleport>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const showModal = ref(false);
return {
showModal,
};
},
};
</script>
<style>
.modal {
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background: white;
padding: 20px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
</style>
在这个例子中,两个模态框分别被传送到 #modal-container-1
和 #modal-container-2
。
总结
Teleport
是 Vue 3 中一个非常有用的特性,它允许你将组件的某部分内容“传送”到 DOM 中的其他位置。这在处理模态框、弹出框、通知等场景时非常有用,可以避免 CSS 或 z-index 的问题。
- 基本用法:使用
<teleport to="target">
将内容传送到指定的目标位置。 - 动态目标:
to
属性可以绑定到一个动态的 DOM 元素。 - 多个目标:可以在同一个组件中使用多个
<teleport>
,并将它们传送到不同的目标位置。
通过 Teleport
,你可以更灵活地控制组件的渲染位置,而不必改变组件的逻辑结构。
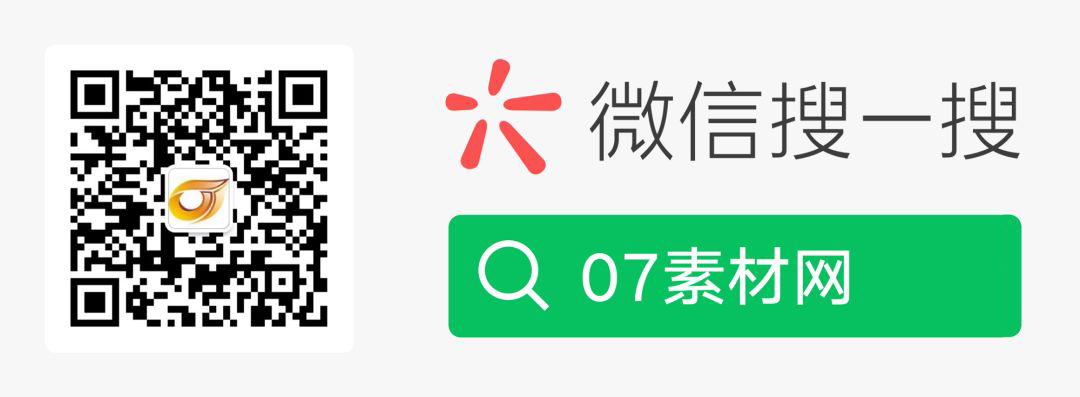