
在当今前端开发领域,Vue.js因其简洁的API和灵活的架构而广受欢迎。然而,随着项目规模的增长,打包后的文件体积往往会急剧膨胀,导致应用加载速度变慢,影响用户体验。本文将全面探讨Vue项目打包优化的各种策略和技术,帮助开发者构建更高效的Vue应用。
二、Webpack基础配置优化
生产模式与开发模式分离
// vue.config.js module.exports = { configureWebpack: config => { if (process.env.NODE_ENV === 'production') { // 生产环境特定配置 } else { // 开发环境特定配置 } } }
设置正确的mode
module.exports = { mode: process.env.NODE_ENV === 'production' ? 'production' : 'development' }
配置resolve.alias
const path = require('path') module.exports = { configureWebpack: { resolve: { alias: { '@': path.resolve(__dirname, 'src'), 'components': path.resolve(__dirname, 'src/components') } } } }
三、代码分割与懒加载
路由级代码分割
const Home = () => import(/* webpackChunkName: "home" */ './views/Home.vue') const About = () => import(/* webpackChunkName: "about" */ './views/About.vue')
组件级懒加载
export default { components: { 'my-component': () => import('./components/MyComponent.vue') } }
Webpack的splitChunks配置
module.exports = { configureWebpack: { optimization: { splitChunks: { chunks: 'all', cacheGroups: { vendor: { test: /[\/]node_modules[\/]/, name: 'vendors', chunks: 'all' } } } } } }
四、Tree Shaking与副作用处理
确保ES模块导入
import { debounce } from 'lodash-es' // 而不是 import _ from 'lodash'
package.json中的sideEffects配置
{ "sideEffects": [ "*.css", "*.scss", "*.vue" ] }
PurgeCSS优化
const PurgecssPlugin = require('purgecss-webpack-plugin') const glob = require('glob-all') const path = require('path') module.exports = { configureWebpack: { plugins: [ new PurgecssPlugin({ paths: glob.sync([ path.join(__dirname, './src/**/*.vue'), path.join(__dirname, './public/index.html') ]), whitelist: ['html', 'body'] }) ] } }
五、第三方库优化策略
CDN引入大型库
// vue.config.js module.exports = { configureWebpack: { externals: { vue: 'Vue', 'vue-router': 'VueRouter', axios: 'axios' } } }
<!-- public/index.html --> <script src="https://cdn.jsdelivr.net/npm/vue@2.6.12/dist/vue.runtime.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/vue-router@3.4.9/dist/vue-router.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/axios@0.21.1/dist/axios.min.js"></script>
按需引入组件库
import { Button, Select } from 'element-ui' Vue.use(Button) Vue.use(Select)
使用轻量级替代方案
- 使用day.js代替moment.js
- 使用lodash-es并按需引入
六、构建产物分析与优化
Webpack Bundle Analyzer
const BundleAnalyzerPlugin = require('webpack-bundle-analyzer').BundleAnalyzerPlugin module.exports = { configureWebpack: { plugins: [ new BundleAnalyzerPlugin() ] } }
Gzip压缩
const CompressionWebpackPlugin = require('compression-webpack-plugin') module.exports = { configureWebpack: { plugins: [ new CompressionWebpackPlugin({ filename: '[path].gz[query]', algorithm: 'gzip', test: /.(js|css|json|txt|html|ico|svg)(?.*)?$/i, threshold: 10240, minRatio: 0.8 }) ] } }
图片优化
module.exports = { chainWebpack: config => { config.module .rule('images') .use('image-webpack-loader') .loader('image-webpack-loader') .options({ mozjpeg: { progressive: true, quality: 65 }, optipng: { enabled: false }, pngquant: { quality: [0.65, 0.90], speed: 4 }, gifsicle: { interlaced: false }, webp: { quality: 75 } }) } }
七、运行时性能优化
v-for使用key
<template v-for="item in items"> <div :key="item.id">{{ item.text }}</div> </template>
避免v-if和v-for一起使用
<!-- 不推荐 --> <div v-for="item in items" v-if="item.isActive"></div> <!-- 推荐 --> <div v-for="item in activeItems"></div>
合理使用计算属性
computed: { filteredItems() { return this.items.filter(item => item.isActive) } }
函数式组件
export default { functional: true, props: ['item'], render(h, { props }) { return h('div', props.item.text) } }
八、部署优化
HTTP/2服务器推送
server { listen 443 ssl http2; http2_push /static/js/app.js; http2_push /static/css/app.css; }
缓存策略
location ~* .(js|css|png|jpg|jpeg|gif|ico|svg)$ { expires 1y; add_header Cache-Control "public, no-transform"; }
Brotli压缩
brotli on; brotli_comp_level 6; brotli_types text/plain text/css application/json application/javascript text/xml application/xml application/xml+rss text/javascript;
九、监控与持续优化
性能指标监控
// 使用web-vitals库 import { getCLS, getFID, getLCP } from 'web-vitals' getCLS(console.log) getFID(console.log) getLCP(console.log)
错误监控
Vue.config.errorHandler = function (err, vm, info) { // 发送错误到监控服务 logErrorToService(err, vm, info) }
A/B测试优化
// 使用Google Optimize或其他A/B测试工具 window.dataLayer = window.dataLayer || [] function gtag() { dataLayer.push(arguments) } gtag('js', new Date()) gtag('config', 'GA_MEASUREMENT_ID', { optimize_id: 'OPT_CONTAINER_ID' })
十、总结
Vue项目打包优化是一个系统工程,需要从多个维度进行考虑和实施。本文介绍的优化策略涵盖了从Webpack配置到运行时性能,从代码分割到部署优化的各个方面。实际项目中,应根据具体情况选择合适的优化方案,并通过性能监控持续改进。
记住,优化是一个持续的过程,而不是一次性的任务。随着项目的发展和技术的演进,新的优化机会将不断出现。保持对性能的关注,定期审查和优化你的Vue应用,将有助于为用户提供最佳的体验。
学在每日,进无止境!更多精彩内容请关注微信公众号。
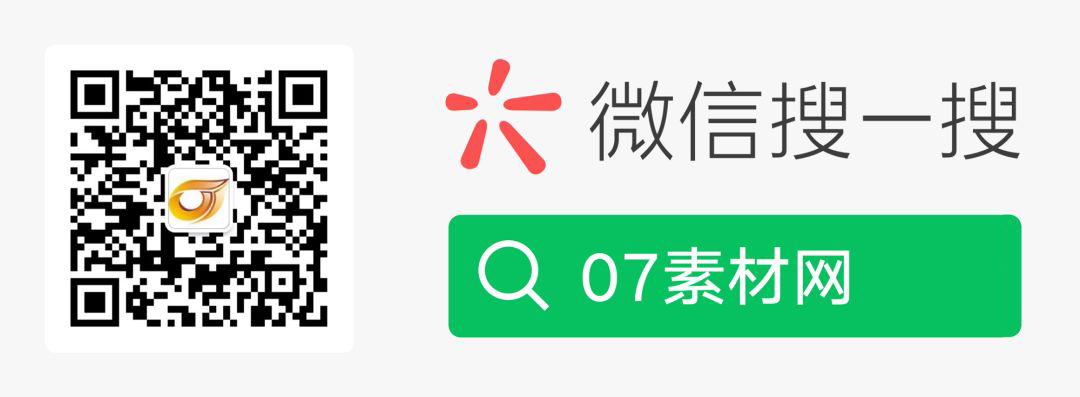
原文出处:
内容由AI生成仅供参考,请勿使用于商业用途。如若转载请注明原文及出处。
出处地址:http://www.07sucai.com/tech/907.html
版权声明:本文来源地址若非本站均为转载,若侵害到您的权利,请及时联系我们,我们会在第一时间进行处理。