
在 Vue 3 中,reactive
是用于创建响应式对象的工具。它适用于复杂数据结构(如对象、数组等)。以下是使用 reactive
创建响应式对象的详细步骤和示例:
1. 引入 reactive
首先,你需要在 Vue 组件中引入 reactive
函数。
import { reactive } from 'vue';
2. 创建响应式对象
使用 reactive
函数创建一个响应式对象。reactive
返回一个响应式代理对象。
const state = reactive({
count: 0,
name: 'Vue 3'
});
3. 访问和修改数据
直接访问和修改 reactive
创建的对象的属性。
console.log(state.count); // 访问数据
state.count++; // 修改数据
4. 在模板中使用
在模板中,你可以直接使用 reactive
创建的对象的属性。
<template>
<div>
<p>{{ state.count }}</p>
<p>{{ state.name }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script>
import { reactive } from 'vue';
export default {
setup() {
const state = reactive({
count: 0,
name: 'Vue 3'
});
function increment() {
state.count++;
}
return {
state,
increment
};
}
};
</script>
5. 在组合式 API 中使用
在组合式 API 中,reactive
通常用于在 setup
函数中创建响应式对象。
import { reactive } from 'vue';
export default {
setup() {
const state = reactive({
count: 0,
name: 'Vue 3'
});
function increment() {
state.count++;
}
return {
state,
increment
};
}
};
6. 在 <script setup>
语法中使用
如果你使用 <script setup>
语法,可以更简洁地使用 reactive
。
<template>
<div>
<p>{{ state.count }}</p>
<p>{{ state.name }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script setup>
import { reactive } from 'vue';
const state = reactive({
count: 0,
name: 'Vue 3'
});
function increment() {
state.count++;
}
</script>
7. 处理嵌套对象
reactive
可以处理嵌套对象,嵌套的对象也会被转换为响应式对象。
const state = reactive({
user: {
name: 'John',
age: 30
},
list: [1, 2, 3]
});
console.log(state.user.name); // 访问嵌套对象属性
state.user.age++; // 修改嵌套对象属性
console.log(state.list[0]); // 访问数组元素
state.list.push(4); // 修改数组
8. 在模板中使用嵌套对象
在模板中,你可以直接使用 reactive
创建的嵌套对象的属性。
<template>
<div>
<p>{{ state.user.name }} is {{ state.user.age }} years old.</p>
<ul>
<li v-for="item in state.list" :key="item">{{ item }}</li>
</ul>
</div>
</template>
<script setup>
import { reactive } from 'vue';
const state = reactive({
user: {
name: 'John',
age: 30
},
list: [1, 2, 3]
});
</script>
9. 解构和响应性
解构 reactive
对象时,响应性会丢失。需要使用 toRefs
来保持响应性。
import { reactive, toRefs } from 'vue';
const state = reactive({
count: 0,
name: 'Vue 3'
});
const { count, name } = toRefs(state); // 使用 toRefs 保持响应性
console.log(count.value); // 访问数据
count.value++; // 修改数据
总结
- 使用
reactive
创建响应式对象时,直接访问和修改对象的属性。 - 在模板中,可以直接使用
reactive
创建的对象的属性。 reactive
适用于复杂数据结构(如对象、数组等),并且可以处理嵌套对象。- 在组合式 API 和
<script setup>
语法中,reactive
是创建响应式对象的常用工具。 - 解构
reactive
对象时,使用toRefs
来保持响应性。
学在每日,进无止境!更多精彩内容请关注微信公众号。
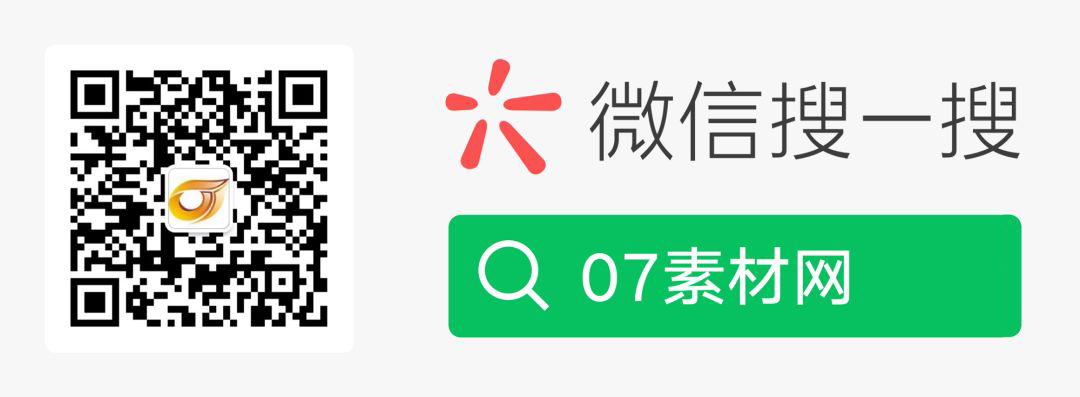
原文出处:
内容由AI生成仅供参考,请勿使用于商业用途。如若转载请注明原文及出处。
出处地址:http://www.07sucai.com/tech/864.html
版权声明:本文来源地址若非本站均为转载,若侵害到您的权利,请及时联系我们,我们会在第一时间进行处理。