
开发一个 Pinia 插件非常简单。Pinia 的插件机制允许你扩展 Store 的功能,例如添加全局状态、监听状态变化、扩展 Store 的方法等。以下是开发 Pinia 插件的详细步骤:
1. 插件的基本结构
Pinia 插件是一个函数,接收一个 PiniaPluginContext
参数,该参数包含以下属性:
store
:当前 Store 实例。app
:Vue 应用实例。pinia
:Pinia 实例。options
:定义 Store 时的选项。
示例:基本插件结构
import { PiniaPluginContext } from 'pinia';
export function myPlugin(context: PiniaPluginContext) {
// 插件逻辑
}
2. 在插件中访问 Store
你可以通过 context.store
访问当前 Store 实例,并对其进行扩展。
示例:访问 Store
import { PiniaPluginContext } from 'pinia';
export function myPlugin(context: PiniaPluginContext) {
console.log(`Store ${context.store.$id} initialized`);
}
3. 监听状态变化
通过 context.store.$subscribe
方法,可以监听 Store 的状态变化。
示例:监听状态变化
import { PiniaPluginContext } from 'pinia';
export function myPlugin(context: PiniaPluginContext) {
context.store.$subscribe((mutation, state) => {
console.log(`Store ${context.store.$id} changed:`, state);
});
}
4. 扩展 Store 的功能
你可以通过 context.store
扩展 Store 的功能,例如添加新的方法或属性。
示例:扩展 Store 功能
import { PiniaPluginContext } from 'pinia';
export function myPlugin(context: PiniaPluginContext) {
context.store.$actions.customAction = function () {
console.log('Custom action executed');
};
}
5. 添加全局状态
你可以通过 context.store.$state
添加全局状态。
示例:添加全局状态
import { PiniaPluginContext } from 'pinia';
export function myPlugin(context: PiniaPluginContext) {
context.store.$state.global = {
loading: false,
error: null,
};
context.store.$actions.setLoading = function (loading: boolean) {
this.global.loading = loading;
};
context.store.$actions.setError = function (error: string | null) {
this.global.error = error;
};
}
6. 使用插件
在创建 Pinia 实例后,使用 pinia.use
方法注册插件。
示例:使用插件
import { createPinia } from 'pinia';
import { myPlugin } from './plugins/myPlugin';
const pinia = createPinia();
pinia.use(myPlugin);
export default pinia;
7. 完整的插件示例
以下是一个完整的 Pinia 插件示例,该插件用于记录 Store 的状态变化并添加一个自定义方法。
示例:完整的插件
import { PiniaPluginContext } from 'pinia';
export function myPlugin(context: PiniaPluginContext) {
// 在 Store 初始化时执行
console.log(`Store ${context.store.$id} initialized`);
// 监听状态变化
context.store.$subscribe((mutation, state) => {
console.log(`Store ${context.store.$id} changed:`, state);
});
// 扩展 Store 的功能
context.store.$actions.customAction = function () {
console.log('Custom action executed');
};
// 添加全局状态
context.store.$state.global = {
loading: false,
error: null,
};
context.store.$actions.setLoading = function (loading: boolean) {
this.global.loading = loading;
};
context.store.$actions.setError = function (error: string | null) {
this.global.error = error;
};
}
使用插件
import { createPinia } from 'pinia';
import { myPlugin } from './plugins/myPlugin';
const pinia = createPinia();
pinia.use(myPlugin);
export default pinia;
8. 插件的类型安全
如果你使用 TypeScript,可以为插件添加类型注解,确保类型安全。
示例:类型安全的插件
import { PiniaPluginContext } from 'pinia';
declare module 'pinia' {
export interface PiniaCustomProperties {
customAction: () => void;
setLoading: (loading: boolean) => void;
setError: (error: string | null) => void;
global: {
loading: boolean;
error: string | null;
};
}
}
export function myPlugin(context: PiniaPluginContext) {
context.store.$actions.customAction = function () {
console.log('Custom action executed');
};
context.store.$state.global = {
loading: false,
error: null,
};
context.store.$actions.setLoading = function (loading: boolean) {
this.global.loading = loading;
};
context.store.$actions.setError = function (error: string | null) {
this.global.error = error;
};
}
总结
开发 Pinia 插件的步骤如下:
- 定义插件函数:接收
PiniaPluginContext
参数。 - 访问 Store:通过
context.store
访问当前 Store 实例。 - 监听状态变化:使用
context.store.$subscribe
监听状态变化。 - 扩展 Store 功能:通过
context.store
添加新的方法或属性。 - 添加全局状态:通过
context.store.$state
添加全局状态。 - 使用插件:在创建 Pinia 实例后,使用
pinia.use
注册插件。 - 类型安全:使用 TypeScript 为插件添加类型注解。
通过以上步骤,你可以开发出功能强大的 Pinia 插件,满足各种复杂的状态管理需求。
学在每日,进无止境!更多精彩内容请关注微信公众号。
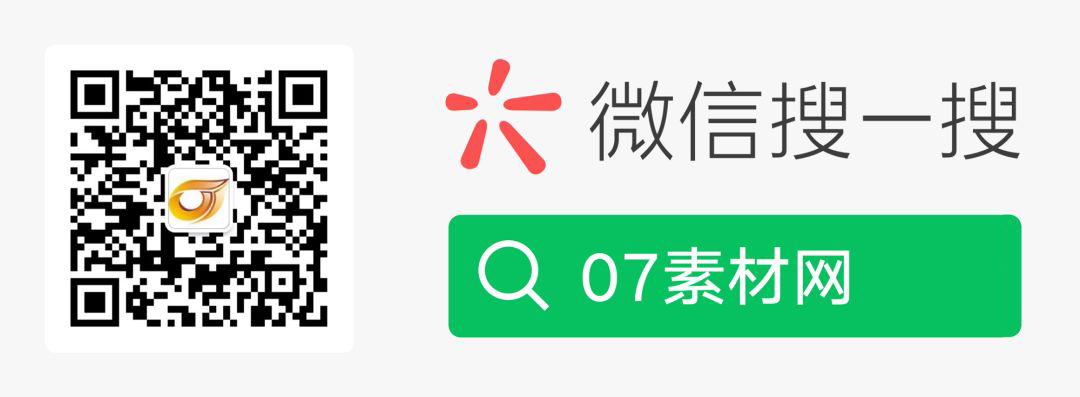
原文出处:
内容由AI生成仅供参考,请勿使用于商业用途。如若转载请注明原文及出处。
出处地址:http://www.07sucai.com/tech/841.html
版权声明:本文来源地址若非本站均为转载,若侵害到您的权利,请及时联系我们,我们会在第一时间进行处理。