
尽管 Pinia 本身已经是一个高效的状态管理库,但在大型应用中,仍然可以通过一些优化技巧进一步提升性能。以下是一些优化 Pinia 性能的常见方法:
1. 按需加载 Store
将 Store 拆分为多个独立的模块,并按需加载。只有在需要时才初始化 Store,减少初始加载时的资源消耗。
示例:动态加载 Store
// 在组件中动态加载 Store
import { defineAsyncComponent } from 'vue';
const UserProfile = defineAsyncComponent(() =>
import('@/stores/userProfile').then((module) => module.useUserProfileStore())
);
2. 减少不必要的状态更新
确保只有实际使用的状态发生变化时,才会触发组件的重新渲染。可以通过以下方式实现:
- 使用
storeToRefs
解构 Store,避免直接解构导致失去响应性。 - 在组件中使用
computed
属性,确保只有依赖的状态变化时才会重新计算。
示例:使用 storeToRefs
import { storeToRefs } from 'pinia';
import { useUserStore } from '@/stores/user';
export default {
setup() {
const userStore = useUserStore();
const { name, age } = storeToRefs(userStore); // 保持响应性
return {
name,
age,
};
},
};
3. 避免在 Store 中保存大量数据
如果 Store 中保存了大量数据,可能会导致内存占用过高和性能下降。可以通过以下方式优化:
- 只保存必要的数据,避免冗余。
- 使用分页或懒加载技术,按需加载数据。
示例:分页加载数据
// stores/user.ts
import { defineStore } from 'pinia';
export const useUserStore = defineStore('user', {
state: () => ({
users: [] as any[],
page: 1,
loading: false,
}),
actions: {
async fetchNextPage() {
this.loading = true;
const response = await fetch(`https://jsonplaceholder.typicode.com/users?_page=${this.page}&_limit=10`);
const data = await response.json();
this.users = [...this.users, ...data];
this.page++;
this.loading = false;
},
},
});
4. 使用缓存
对于不经常变化的数据,可以使用缓存机制,减少重复请求和数据计算。
示例:使用缓存
// stores/user.ts
import { defineStore } from 'pinia';
export const useUserStore = defineStore('user', {
state: () => ({
user: null as any,
cache: {} as Record<number, any>, // 缓存用户数据
}),
actions: {
async fetchUser(userId: number) {
if (this.cache[userId]) {
this.user = this.cache[userId]; // 使用缓存数据
return;
}
const response = await fetch(`https://jsonplaceholder.typicode.com/users/${userId}`);
const data = await response.json();
this.user = data;
this.cache[userId] = data; // 缓存数据
},
},
});
5. 优化 Actions
确保 actions
中的逻辑高效,避免不必要的计算或请求。可以将复杂的逻辑拆分为多个小的 actions
,便于复用和优化。
示例:拆分 Actions
// stores/user.ts
import { defineStore } from 'pinia';
export const useUserStore = defineStore('user', {
state: () => ({
user: null as any,
loading: false,
}),
actions: {
async fetchUser(userId: number) {
this.loading = true;
const response = await this.fetchUserData(userId);
this.user = response;
this.loading = false;
},
async fetchUserData(userId: number) {
const response = await fetch(`https://jsonplaceholder.typicode.com/users/${userId}`);
return response.json();
},
},
});
6. 使用插件优化
Pinia 支持插件机制,可以通过插件优化状态管理流程。例如,可以开发一个插件来记录状态变化,帮助分析性能瓶颈。
示例:性能分析插件
// plugins/performance.ts
import { PiniaPluginContext } from 'pinia';
export function performancePlugin(context: PiniaPluginContext) {
context.store.$subscribe((mutation, state) => {
console.time(`Store ${context.store.$id} update`);
console.timeEnd(`Store ${context.store.$id} update`);
});
}
// 使用插件
import { createPinia } from 'pinia';
import { performancePlugin } from './plugins/performance';
const pinia = createPinia();
pinia.use(performancePlugin);
7. 避免频繁的状态更新
如果某个状态频繁变化(如滚动位置),可以考虑使用防抖(debounce)或节流(throttle)技术,减少状态更新的频率。
示例:使用防抖
import { defineStore } from 'pinia';
import { debounce } from 'lodash-es';
export const useScrollStore = defineStore('scroll', {
state: () => ({
scrollPosition: 0,
}),
actions: {
updateScrollPosition: debounce(function (this: any, position: number) {
this.scrollPosition = position;
}, 100), // 100ms 防抖
},
});
8. 使用 shallowRef
或 shallowReactive
如果某些状态不需要深度监听,可以使用 shallowRef
或 shallowReactive
来减少响应式系统的开销。
示例:使用 shallowRef
import { defineStore } from 'pinia';
import { shallowRef } from 'vue';
export const useUserStore = defineStore('user', {
state: () => ({
user: shallowRef(null), // 浅层响应式
}),
});
9. 优化 Devtools 集成
在生产环境中,可以禁用 Vue Devtools,减少性能开销。
示例:禁用 Devtools
import { createPinia } from 'pinia';
const pinia = createPinia();
pinia.use(({ store }) => {
if (process.env.NODE_ENV === 'production') {
store._devtools = false; // 禁用 Devtools
}
});
总结
优化 Pinia 性能的常见方法包括:
- 按需加载 Store:减少初始加载时的资源消耗。
- 减少不必要的状态更新:使用
storeToRefs
和computed
。 - 避免保存大量数据:使用分页或懒加载技术。
- 使用缓存:减少重复请求和数据计算。
- 优化 Actions:拆分复杂逻辑,避免不必要的计算。
- 使用插件优化:开发性能分析插件。
- 避免频繁的状态更新:使用防抖或节流技术。
- 使用
shallowRef
或shallowReactive
:减少响应式系统的开销。 - 优化 Devtools 集成:在生产环境中禁用 Devtools。
通过这些优化技巧,可以进一步提升 Pinia 的性能,特别是在大型应用中。
学在每日,进无止境!更多精彩内容请关注微信公众号。
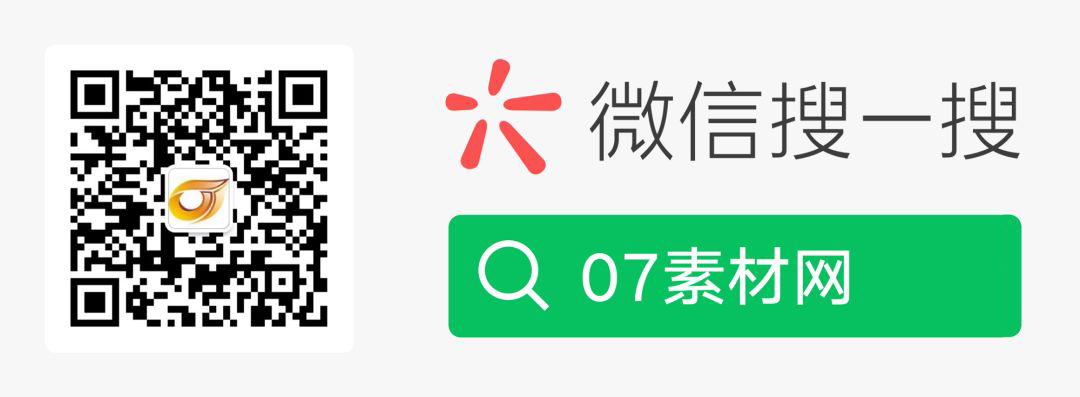
原文出处:
内容由AI生成仅供参考,请勿使用于商业用途。如若转载请注明原文及出处。
出处地址:http://www.07sucai.com/tech/837.html
版权声明:本文来源地址若非本站均为转载,若侵害到您的权利,请及时联系我们,我们会在第一时间进行处理。