在 Vue 3 中使用 Vuex 进行状态管理与 Vue 2 类似,但由于 Vue 3 引入了 Composition API,因此在使用 Vuex 时也有一些新的方式和优化。以下是详细的使用方法:
1. 安装 Vuex
首先,确保你已经安装了 Vuex。如果尚未安装,可以通过以下命令安装:
npm install vuex@next
2. 创建 Vuex Store
在 Vue 3 中创建 Vuex Store 的方式与 Vue 2 相同。以下是一个简单的 Vuex Store 示例:
// store/index.js
import { createStore } from 'vuex';
const store = createStore({
state: {
count: 0,
},
mutations: {
increment(state) {
state.count ;
},
setCount(state, value) {
state.count = value;
},
},
actions: {
incrementAsync({ commit }) {
setTimeout(() => {
commit('increment');
}, 1000);
},
},
getters: {
doubleCount(state) {
return state.count * 2;
},
},
});
export default store;
3. 在 Vue 3 应用中引入 Vuex Store
在 Vue 3 应用中,通过 app.use(store)
将 Vuex Store 注入到应用中:
// main.js
import { createApp } from 'vue';
import App from './App.vue';
import store from './store';
const app = createApp(App);
app.use(store);
app.mount('#app');
4. 在组件中使用 Vuex
在 Vue 3 中,可以通过 Options API 或 Composition API 使用 Vuex。
Options API 中使用 Vuex
在 Options API 中,可以通过 this.$store
访问 Vuex Store。
<template>
<div>
<p>Count: {{ count }}</p>
<p>Double Count: {{ doubleCount }}</p>
<button @click="increment">Increment</button>
<button @click="incrementAsync">Increment Async</button>
</div>
</template>
<script>
export default {
computed: {
count() {
return this.$store.state.count;
},
doubleCount() {
return this.$store.getters.doubleCount;
},
},
methods: {
increment() {
this.$store.commit('increment');
},
incrementAsync() {
this.$store.dispatch('incrementAsync');
},
},
};
</script>
Composition API 中使用 Vuex
在 Composition API 中,可以使用 useStore
钩子访问 Vuex Store。
<template>
<div>
<p>Count: {{ count }}</p>
<p>Double Count: {{ doubleCount }}</p>
<button @click="increment">Increment</button>
<button @click="incrementAsync">Increment Async</button>
</div>
</template>
<script>
import { computed } from 'vue';
import { useStore } from 'vuex';
export default {
setup() {
const store = useStore();
const count = computed(() => store.state.count);
const doubleCount = computed(() => store.getters.doubleCount);
function increment() {
store.commit('increment');
}
function incrementAsync() {
store.dispatch('incrementAsync');
}
return {
count,
doubleCount,
increment,
incrementAsync,
};
},
};
</script>
5. 模块化 Vuex Store
在大型应用中,通常会将 Vuex Store 拆分为多个模块。以下是一个模块化的 Vuex Store 示例:
定义模块
// store/modules/counter.js
const counterModule = {
namespaced: true,
state: {
count: 0,
},
mutations: {
increment(state) {
state.count ;
},
},
actions: {
incrementAsync({ commit }) {
setTimeout(() => {
commit('increment');
}, 1000);
},
},
getters: {
doubleCount(state) {
return state.count * 2;
},
},
};
export default counterModule;
注册模块
// store/index.js
import { createStore } from 'vuex';
import counterModule from './modules/counter';
const store = createStore({
modules: {
counter: counterModule,
},
});
export default store;
在组件中使用模块
在组件中使用模块化的 Vuex Store 时,需要指定模块的命名空间。
<template>
<div>
<p>Count: {{ count }}</p>
<p>Double Count: {{ doubleCount }}</p>
<button @click="increment">Increment</button>
<button @click="incrementAsync">Increment Async</button>
</div>
</template>
<script>
import { computed } from 'vue';
import { useStore } from 'vuex';
export default {
setup() {
const store = useStore();
const count = computed(() => store.state.counter.count);
const doubleCount = computed(() => store.getters['counter/doubleCount']);
function increment() {
store.commit('counter/increment');
}
function incrementAsync() {
store.dispatch('counter/incrementAsync');
}
return {
count,
doubleCount,
increment,
incrementAsync,
};
},
};
</script>
6. 使用 Vuex 辅助函数
Vuex 提供了一些辅助函数(如 mapState
、mapGetters
、mapMutations
、mapActions
),可以简化在组件中使用 Vuex 的代码。
Options API 中使用辅助函数
<template>
<div>
<p>Count: {{ count }}</p>
<p>Double Count: {{ doubleCount }}</p>
<button @click="increment">Increment</button>
<button @click="incrementAsync">Increment Async</button>
</div>
</template>
<script>
import { mapState, mapGetters, mapMutations, mapActions } from 'vuex';
export default {
computed: {
...mapState('counter', ['count']),
...mapGetters('counter', ['doubleCount']),
},
methods: {
...mapMutations('counter', ['increment']),
...mapActions('counter', ['incrementAsync']),
},
};
</script>
Composition API 中使用辅助函数
在 Composition API 中,可以手动实现类似的功能,或者使用第三方库(如 vuex-composition-helpers
)。
7. 总结
在 Vue 3 中使用 Vuex 进行状态管理的步骤如下:
- 安装 Vuex:通过
npm install vuex@next
安装 Vuex。 - 创建 Vuex Store:使用
createStore
创建 Store,并定义state
、mutations
、actions
和getters
。 - 在应用中引入 Store:通过
app.use(store)
将 Store 注入到应用中。 - 在组件中使用 Store:
- 在 Options API 中,通过
this.$store
访问 Store。 - 在 Composition API 中,使用
useStore
钩子访问 Store。
- 在 Options API 中,通过
- 模块化 Store:将 Store 拆分为多个模块,并在组件中使用命名空间访问模块。
- 使用辅助函数:通过
mapState
、mapGetters
、mapMutations
、mapActions
简化代码。
通过以上步骤,你可以在 Vue 3 中高效地使用 Vuex 进行状态管理。
- - - - - - - 剩余部分未读 - - - - - - -
扫描关注微信公众号获取验证码,阅读全文
你也可以查看我的公众号文章,阅读全文
你还可以登录,阅读全文
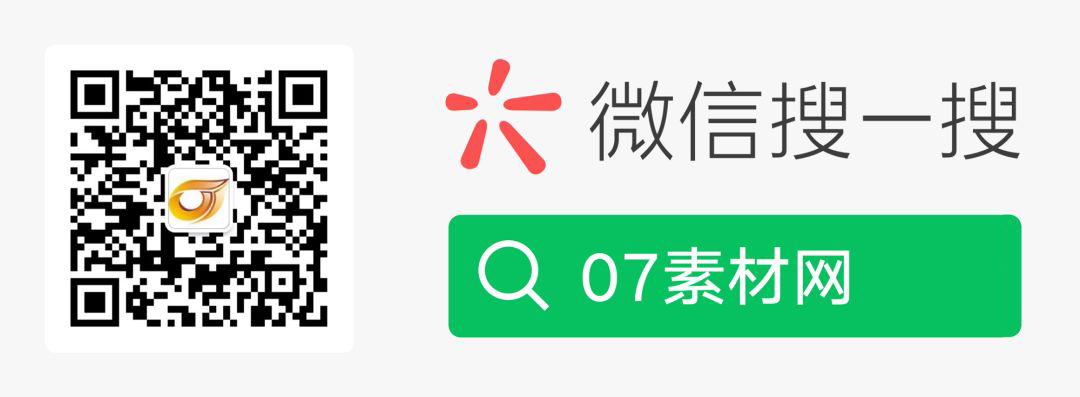
内容由AI生成仅供参考和学习交流,请勿使用于商业用途。
出处地址:http://www.07sucai.com/tech/617.html,如若转载请注明原文及出处。
版权声明:本文来源地址若非本站均为转载,若侵害到您的权利,请及时联系我们,我们会在第一时间进行处理。