
在React中,组件之间的通信是一个常见且重要的概念。组件之间的通信方式有多种,具体选择哪种方式取决于组件之间的关系(如父子关系、兄弟关系、跨级关系等)。以下是几种常见的组件通信方式:
- 父子组件通信
父组件向子组件传递数据(props)
父组件通过props向子组件传递数据。
jsx复制代码// 父组件function ParentComponent() { const data = "Hello from Parent!"; return
;} // 子组件function ChildComponent(props) { return {props.message};} 子组件向父组件传递数据(回调函数) 子组件通过回调函数(props传递的函数)向父组件传递数据。 jsx复制代码// 父组件function ParentComponent() { const handleMessage = (message) => { console.log(message); }; return;} // 子组件function ChildComponent(props) { const sendMessage = () => { props.onSendMessage("Hello from Child!"); }; return ;} - 兄弟组件通信
兄弟组件之间的通信通常通过父组件来作为中介。
jsx复制代码// 父组件function ParentComponent() { const [message, setMessage] = useState(""); const handleMessage = (msg) => { setMessage(msg); }; return ( {props.message};}
- 跨级组件通信
使用React Context
React Context 提供了一种在组件树中传递数据的方法,而不必在每一层手动传递props。
jsx复制代码// 创建一个Contextconst MyContext = React.createContext(); // 提供数据的组件(父组件或更高层的组件)function ParentComponent() { const [value, setValue] = useState("Initial Value"); return ( <MyContext.Provider value={{ value, setValue }}>
</MyContext.Provider> );} // 消费数据的子组件Afunction ChildComponentA() { const { setValue } = useContext(MyContext); return <button onClick={() => setValue("Updated from A")}>Update Value;} // 消费数据的子组件Bfunction ChildComponentB() { const { value } = useContext(MyContext); return {value};} - 使用全局状态管理库(如Redux、MobX) 对于更复杂的应用,全局状态管理库如Redux或MobX可以帮助管理应用的状态,使得组件之间的通信更加清晰和高效。 使用Redux的示例
安装Redux和React-Redux
bash复制代码npm install redux react-redux
创建Redux Store
javascript复制代码// store.jsimport { createStore } from 'redux'; const initialState = { value: "Initial Value" }; function reducer(state = initialState, action) { switch (action.type) { case 'UPDATE_VALUE': return { ...state, value: action.payload }; default: return state; }} const store = createStore(reducer);export default store;
提供Store给应用
jsx复制代码// index.jsimport React from 'react';import ReactDOM from 'react-dom';import { Provider } from 'react-redux';import store from './store';import App from './App'; ReactDOM.render(
连接组件到Redux Store
jsx复制代码// App.jsimport React from 'react';import { useSelector, useDispatch } from 'react-redux'; function ChildComponentA() { const dispatch = useDispatch(); return <button onClick={() => dispatch({ type: 'UPDATE_VALUE', payload: 'Updated from A' })}>Update Value;} function ChildComponentB() { const value = useSelector((state) => state.value); return
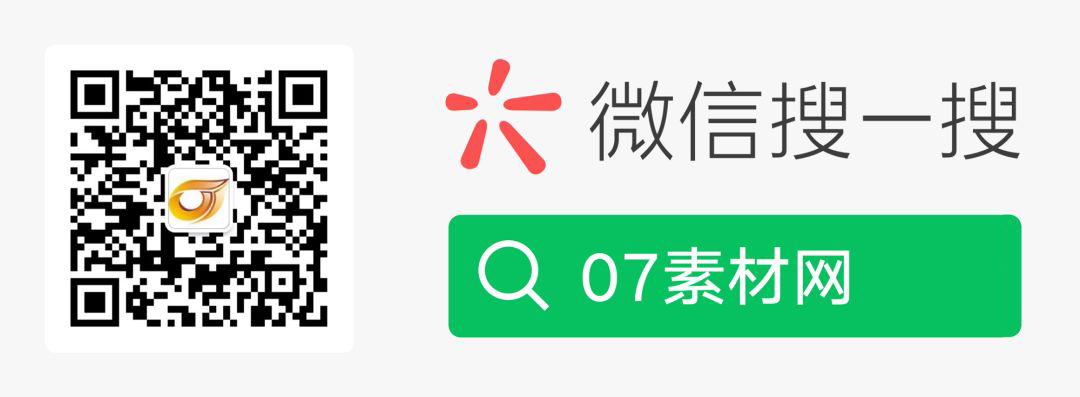