
一、AJAX技术概述
AJAX(Asynchronous JavaScript and XML)是一种创建快速动态网页的技术,它通过在后台与服务器进行少量数据交换,使网页实现异步更新。这意味着可以在不重新加载整个页面的情况下,对网页的某部分进行更新。
传统Web应用与AJAX应用的主要区别在于:
- 传统Web应用:每次用户操作都会导致整个页面刷新
- AJAX应用:只有必要的数据在后台传输,页面局部更新
AJAX的核心优势包括:
- 提升用户体验:减少页面刷新,操作更流畅
- 节省带宽:只传输必要数据而非整个页面
- 减轻服务器负担:减少不必要的完整页面请求
二、AJAX工作原理
AJAX的基本工作原理如下:
- 浏览器中发生事件(页面加载、按钮点击等)
- 创建XMLHttpRequest对象
- 配置请求(方法、URL、异步标志等)
- 发送请求到服务器
- 服务器处理请求并返回响应
- 浏览器通过回调函数处理响应
- 根据需要更新页面内容
这一过程完全由JavaScript控制,实现了真正的异步通信。
三、XMLHttpRequest对象使用
XMLHttpRequest(XHR)是AJAX的基础,所有现代浏览器都支持它。
1. 创建XHR对象
const xhr = new XMLHttpRequest();
2. 配置请求
xhr.open('GET', 'https://api.example.com/data', true); // 异步请求
3. 设置回调函数
xhr.onload = function() {
if (xhr.status >= 200 && xhr.status < 300) {
console.log('成功响应:', xhr.responseText);
} else {
console.error('请求失败:', xhr.statusText);
}
};
xhr.onerror = function() {
console.error('请求出错');
};
4. 发送请求
xhr.send();
5. 发送POST请求示例
const xhr = new XMLHttpRequest();
xhr.open('POST', 'https://api.example.com/submit', true);
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.onload = function() {
// 处理响应
};
const data = JSON.stringify({ username: 'example', password: '123456' });
xhr.send(data);
四、Fetch API的使用
Fetch API是现代浏览器提供的更简洁的AJAX替代方案,基于Promise实现。
1. 基本GET请求
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('网络响应不正常');
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('请求失败:', error));
2. POST请求示例
fetch('https://api.example.com/submit', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ username: 'example', password: '123456' })
})
.then(response => response.json())
.then(data => console.log('成功:', data))
.catch(error => console.error('错误:', error));
3. Fetch API的优势
- 更简洁的语法
- 基于Promise,避免回调地狱
- 更好的错误处理机制
- 内置对JSON的支持
五、处理不同数据格式
1. JSON数据
// 发送JSON
const data = { key: 'value' };
fetch(url, {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(data)
});
// 接收JSON
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
2. FormData
const formData = new FormData();
formData.append('username', 'user1');
formData.append('avatar', fileInput.files[0]);
fetch(url, {
method: 'POST',
body: formData
});
3. URL编码数据
const data = 'name=John&age=30';
fetch(url, {
method: 'POST',
headers: { 'Content-Type': 'application/x-www-form-urlencoded' },
body: data
});
六、错误处理与调试
1. XHR错误处理
xhr.onerror = function() {
// 网络错误
};
xhr.onabort = function() {
// 请求被取消
};
xhr.ontimeout = function() {
// 请求超时
};
2. Fetch错误处理
fetch(url)
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
return response.json();
})
.catch(error => {
console.error('请求出现问题:', error);
});
3. 调试技巧
- 使用浏览器开发者工具查看网络请求
- 检查请求头和响应头
- 验证请求方法和URL是否正确
- 检查跨域问题(CORS)
七、AJAX最佳实践
添加加载状态:在请求期间显示加载指示器
function toggleLoader(show) { document.getElementById('loader').style.display = show ? 'block' : 'none'; } toggleLoader(true); fetch(url) .then(response => response.json()) .then(data => { // 处理数据 }) .finally(() => toggleLoader(false));
超时处理:为请求设置合理超时
// XHR xhr.timeout = 5000; // 5秒 xhr.ontimeout = function() { /* 处理超时 */ }; // Fetch + AbortController const controller = new AbortController(); const timeoutId = setTimeout(() => controller.abort(), 5000); fetch(url, { signal: controller.signal }) .then(response => response.json()) .then(data => { clearTimeout(timeoutId); // 处理数据 }) .catch(error => { if (error.name === 'AbortError') { console.log('请求超时'); } });
安全考虑:
- 始终验证和清理服务器返回的数据
- 使用HTTPS保护敏感数据
- 实施CSRF保护措施
性能优化:
- 减少不必要的请求
- 使用缓存策略
- 压缩传输数据
八、现代AJAX开发
随着前端生态的发展,AJAX技术也在不断演进:
async/await语法:使异步代码更易读
async function fetchData() { try { const response = await fetch(url); if (!response.ok) throw new Error('请求失败'); const data = await response.json(); console.log(data); } catch (error) { console.error('出错:', error); } }
Axios库:提供更丰富的功能
axios.get(url) .then(response => console.log(response.data)) .catch(error => console.error(error)); axios.post(url, { data }) .then(response => /* 处理响应 */);
WebSocket:对于实时应用,可以考虑WebSocket实现全双工通信
九、常见问题与解决方案
跨域问题(CORS)
- 服务器设置正确的CORS头
- 开发环境中配置代理
- JSONP(仅限于GET请求)
兼容性问题
- 使用polyfill为旧浏览器提供Fetch支持
- 对于非常旧的浏览器,准备好XHR回退方案
SEO考虑
- 确保关键内容不依赖AJAX加载
- 使用服务器端渲染(SSR)或预渲染技术
十、总结
AJAX技术彻底改变了Web应用的开发方式,使前后端分离架构成为可能。通过掌握XMLHttpRequest和Fetch API,开发者可以创建高效、响应迅速的Web应用。随着现代JavaScript的发展,async/await等新特性让异步代码更加清晰易读。
在实际项目中,应根据具体需求选择合适的技术方案,并始终关注性能优化、错误处理和安全性。掌握AJAX技术是成为全栈开发者的重要一步,它连接了前端展示与后端逻辑,是现代Web开发的核心技能之一。
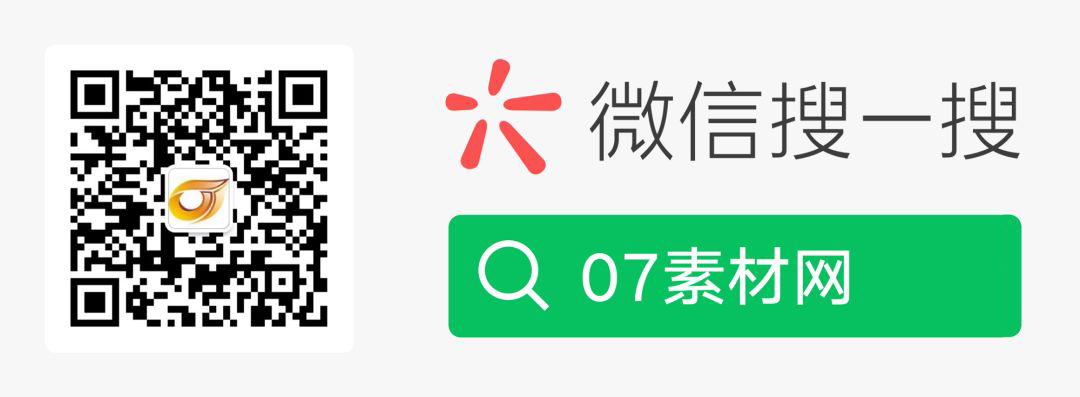